Install Git
The instructions below use Git to get the CS325 code library. If you don't already have Git installed, do so now. This installs Git and, on Windows, the Git Bash shell. Git Bash is a Unix-like shell that you will use to get the code library.
Download and install Common Lisp
Three versions of Common Lisp are recommended for this class. All are free, mature systems.
- LispWorks Personal Edition: This provides an integrated Lisp editing and execution environment for Windows and MacOS (both Intel and Apple Silicon).
- Allegro Common Lisp Express: The Windows version includes an integrated Lisp editing and execution environment. The MacOS version uses a browser-based interface to do this.
- SBCL: ree open-source frequently updated version of Common Lisp with no memory or time limitations. The downside is that a number of additional platform-specific steps are needed to install a Lisp editor, and Windows support is weak.
Scheme is not suitable for this course.
I recommend LispWorks Personal Edition for most students. It is simplest to install and use. Allegro Express is also fine though it has some trouble installing some open-source libraries. While SBCL is most popular with Linux and MacOS users, I don't recommend it for beginners. It has inconsistent support on Windows, doesn't provide a user-friendly Lisp-savvy editor out of the box, and the editor most commonly added is Emacs-based, which is its own learning curve. I am not a regular user of SBCL, so any support for it in this class will have to come from other students.
Allegro: If you plan to use Allegro Express on MacOS with Apple Silicon, i.e., the M1 or M2 chips, be sure to install version 11. A beta version is available on the download page, even though the page title says version 10. For Windows and Intel MacOS, version 10 is fine.
You must use a Lisp-savvy editor that knows how to correctly indent Lisp. If you try to indent by hand, you will get it wrong. I immediately return incorrectly indented Lisp code to be fixed.
Allegro and LispWorks come with a Lisp-savvy editor. SBCL does not. Many SBCL users edit with the SLIME extension for Emacs. Emacs is great but not at all like modern WYSIWYG editors. If you use VS Code, there are some extensions for Common Lisp, but the ones I've tried did not indent Lisp correctly.
Use the extension .lisp
for all
Lisp source files. Some Lisp editors will not indent code in a file properly unless
the file has been saved with a known Lisp extension.
Install Quicklisp
Quicklisp is a Lisp library that makes it easy to download and install other libraries.
Download quicklisp.lisp into some directory that is easy to find.
- Be sure the file is saved with the extension is
lisp
. Browsers often want to save this file asquicklisp.lisp.txt
instead. That won't work. Rename the file if this happened. - Be sure the file doesn't contain HTML. Some browsers add HTML code when you do "Save As..." If this happens, try a different approach to downloading the file. The file should only contain Lisp code.
Start Lisp by double-clicking the icon for the Lisp you installed.
Use File | Load to load quicklisp.lisp. Use Load not Compile.
In the Lisp Listener window, evaluate
(quicklisp-quickstart:install)
This operation will download the files that Quicklisp needs. You will not need to do this again in the future. If there are any errors, ask for help.
Do not call ql:add-to-init-file as described on the Quicklisp page. The instructions below will take care of that part.
Leave Lisp open for now.
Install the CS325 Library
There are a number of Lisp tools used in this class. The source code is on Gitlab. To use them, you install the code as a Quicklisp local project. The following commands will do this on MacOS, Linux, Unix, or Git Bash on Windows.
cd ~/quicklisp/local-projects git clone https://gitlab.com/criesbeck/cs325.git
Check that the library files have been installed.
ls cs325
Now, in Lisp, run the following command:
(load "~/quicklisp/setup.lisp")
This loads Quicklisp. Assuming this works, now do:
(ql:register-local-projects)
This updates a file, ~/quicklisp/local-projects/system-index-txt
that lists the modules in the CS325 library.
Test that this has worked by typing this in Lisp:
(ql:quickload "cs325")
If everything is set up correctly, a number of files will be compiled and loaded. Compiling happens only when a file is added or changed. Future loads will be much quicker.
You will see warnings about some undefined functions. These are functions you will define in exercises. If you see any messages labeled ERROR, stop and check the messages to see if you can determine the problem. Make sure ~/quicklisp/local-projects/cs325/ exists. If you can't figure out the problem, post to Piazza. Include what Lisp and operating system you are using, and copy and paste the exact error message.
Set Up Your Init File
The folder ~/quicklisp/local-projects/c325/init-files
contains several initialization files:
-
clinit.cl
is for Allegro Common Lisp. -
lwinit.lisp
is for Lispworks -
.sbclrc
is for SBCL -- note it starts with a period and has no file extension
You can copy the appropriate init file for your Lisp to your home directory in a terminal shell if you have either Git Bash on Windows, or the MacOS Unix command line tools installed. Git Bash comes with Git. The MacOS Unix command line tools can be installed by running the following command in a terminal window:
xcode-select --install
For more information, see How to Install Command Line Tools in Mac OS X (Without Xcode).
With either Linux, Git Bash on Windows, or the MacOS terminal, install the appropriate init file for your Lisp.
Platform | Command |
---|---|
Allegro |
cp ~/quicklisp/local-projects/cs325/init-files/clinit.cl ~/ |
Lispworks |
cp ~/quicklisp/local-projects/cs325/init-files/lwinit.lisp ~/ |
SBCL |
cp ~/quicklisp/local-projects/cs325/init-files/.sbclrc ~/ |
Exiting Lisp
Time to exit Lisp and see if it starts up properly.
Allegro / LispWorks on Windows: exit with File | Exit.
LispWorks on MacOS: exit with LispWorks Personal | Quit LispWorks Personal.
Allegro on MacOS: in the Allegro browser window, use the Lisp File | Exit menu to stop Lisp, then close the browser tab.
SBCL: evaluate (sb-ext:exit) in Lisp.
Starting Lisp
Here's the normal process for starting Lisp for this class.
Start your Lisp. Allegro and SBCL will start loading the init file automatically.
LispWorks only: The free edition does not auto-load init files.
Use the File | Load menu option to
locate and load the file ~/lwinit.lisp every time you start Lisp.
After loading completes, hit the spacebar
to switch to the Lisp listener window.
Testing Your Setup
If the current Listener package is not CS325-USER, type:
(in-package :CS325-user)
If that gets an error, the 325 code has not been loaded.
Then type:
(critique (defun foo (x) (setq x (+ x 1))))
This calls the Lisp Critic. If all has been set up correctly, several critiques of the above code should be printed.
SBCL on Windows only: If you get an error message running the Lisp Critic, it may be because SBCL can't handle Windows line endings. This affects the Lisp Critic rule file, ~/quicklisp/cs325/lisp-rules.lisp. To fix, you need to convert that file to Unix line endings with some utility, such as dos2unix. Convert that file before continuing. You will need to do this whenever I release updates to the rule file.
Then type
(get-tests)
This should return several lines of names of exercises that you will need to use to check your exercise answers with the Lisp Unit Tester before submitting.
Set Preferences
Some preferences in Lisp need to be set within Lisp.
Lispworks Listener Output
By default, output in the Listener window is underlined in Lispworks to indicate that items printed there are inspectable "marked objects". Since the underlining is pretty ugly, you can turn it off by changing the style for marked objects in the Lispworks Personal | Settings menu on MacOS or Tools | Preferences menu on Windows.
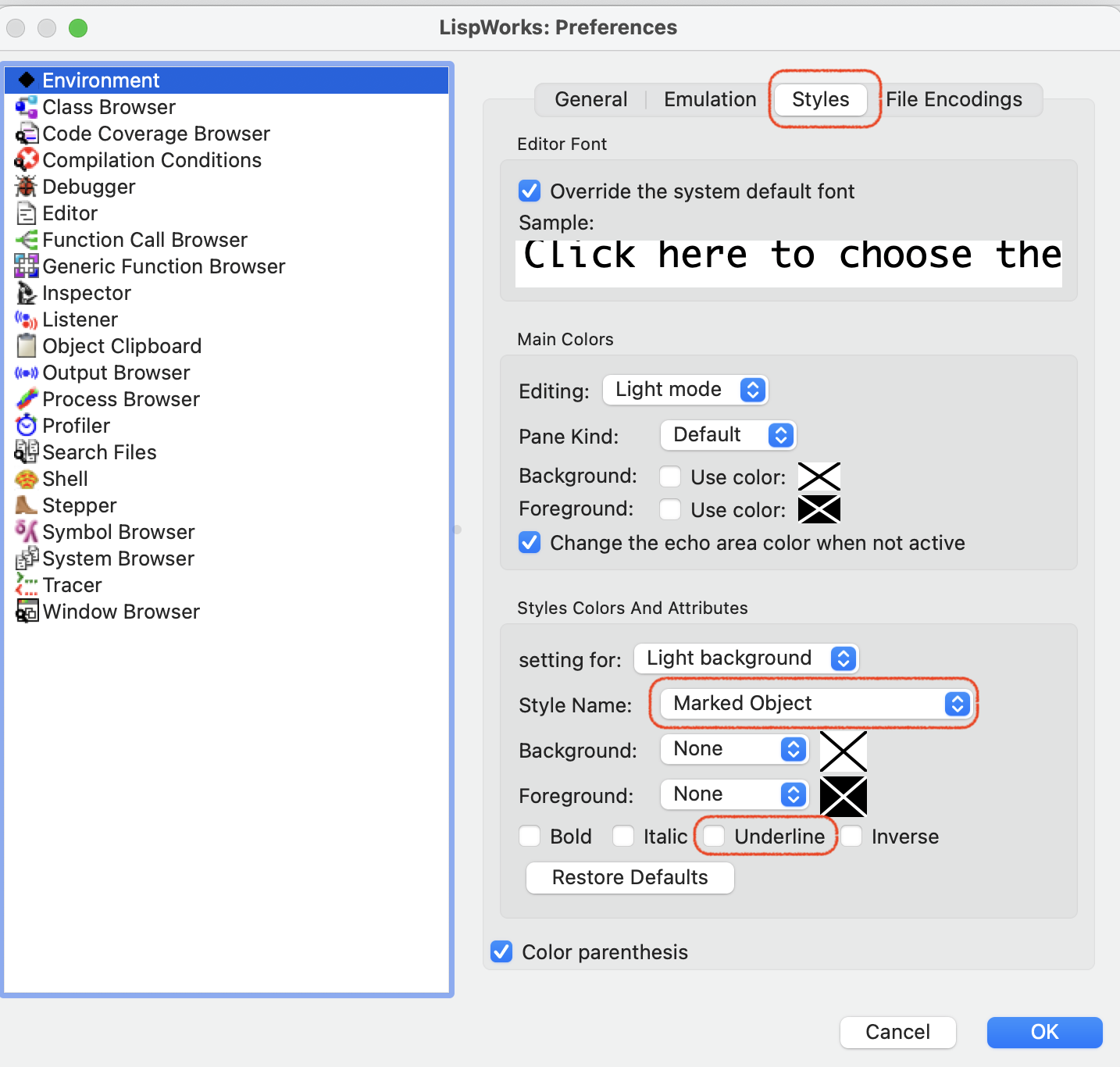
LispWorks Editor Keys
Unless you are a fan of Emacs, you will want to change how keys work in the Lisp editor windows. You can do this in in the Lispworks Personal | Settings menu on MacOS or Tools | Preferences menu on Windows as follows:
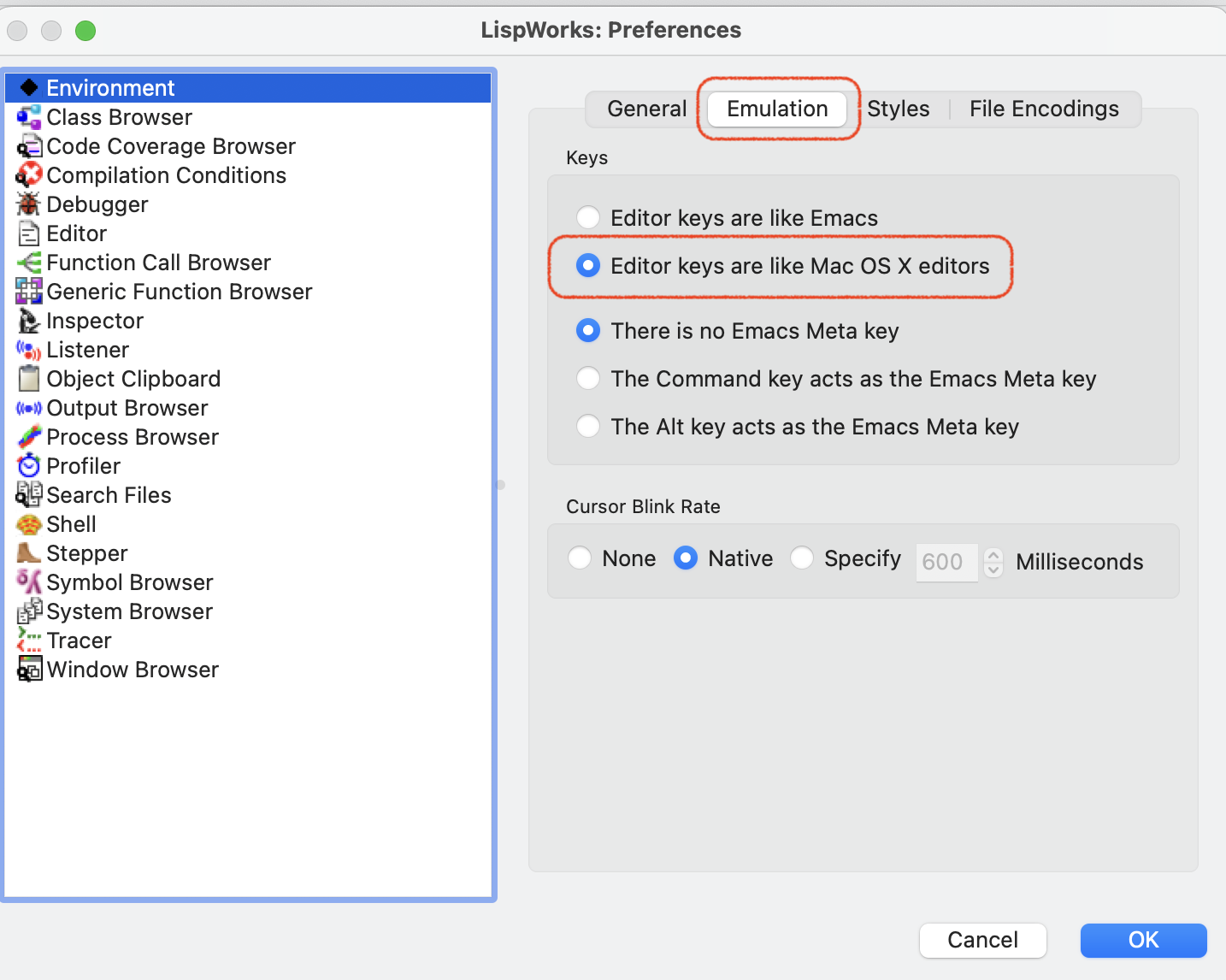
Allegro Initial User Package
You can tell Allegro to switch to the CS325 user package on startup from the Tools | Options... menu:
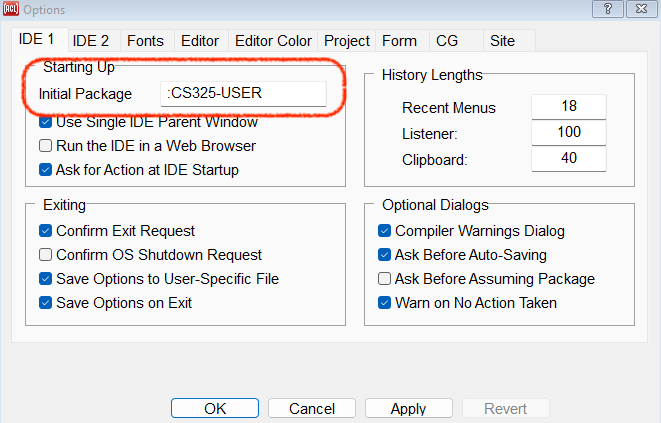
Updating the CS325 library
I will post announcements about updates to the CS325 library on Piazza. When you see these, open a terminal command shell, navigate to the CS325 local project, and use Git to update the code.
cd ~/quicklisp/local-projects/cs325 git pull
The next time you start Lisp, Quicklisp will automatically recompile and load the updated code.
Now exit Lisp and test your setup.
Where to get help
There are many ways to make trivial errors in configuring any language environment, and most of them lead to fairly cryptic messages and behavior.
To get help, post on Piazza.
- Look to see if someone else had the same problem you did, and what answers they got.
- If your problem is a new one, POST your problem. Be specific about what platform you're on, e.g., Windows XP, Linux, ... Copy and paste the error message, if any.
- If you've seen that problem and know the solution, POST the answer. Helpful postings can increase your grade!